In addition to the reading below, you can watch this video for guidance!
If you are unable to download the software due to access issues or hardware restricitons, feel free to use the Online IDE. Follow these Setup instructions to create an account and configure the plugin.
void loop() { // put your main code here, to run repeatedly: digitalWrite(13,HIGH); delay(100); digitalWrite(13,LOW); delay(900); }
<br>
## Troubleshooting
- Is the USB cord plugged in to both the Arduino and the pc/laptop?
- Is the board selected from dropdown in the Arduino IDE?
<br><br>
# Ready for more?
Next we will configire three LEDs to blink in sequence. You will need a single LED and a resistor, let's learn about both of them first. Below is an LED (Light Emitting Diode). Notice that one leg of the LED is longer than the other. The longer leg is the positive (+) side and the shorter leg is the negative (-) side. This is important to remember. Wiring the LED backward wil not allow it to light up (and could destroy it).
<img src=assets/images/LED.jpg width="250" >
You will also need a resistor, which is pictured below. The more resistance provided by the resistor, the less current is allowed to flow to the LED. More current means a brighter light, less current means a dimmer light. If you were to skip the resistor (or use one with too little resistance), you will damage both the LED and the board because the LED would get more current than it can handle and the board would be sending more current than it can support through that pin. The resistor is important to protect the circut from overloading.
Notice the colored bands on the resistor, these indicate the amount of resistance that will be provided. It is admittedly a pretty cryptic system, but resistors are small so it is what it is. You can decode the bands using [online resitor color code calculators](https://circuitdigest.com/calculators/5-band-resistor-color-code-calculator). For our needs we will use a resistor that is between 220 and 330 Ohms. The resistor pictured below is 330 Ohms and has band colors Orange, Orange, Black, Black, Brown.
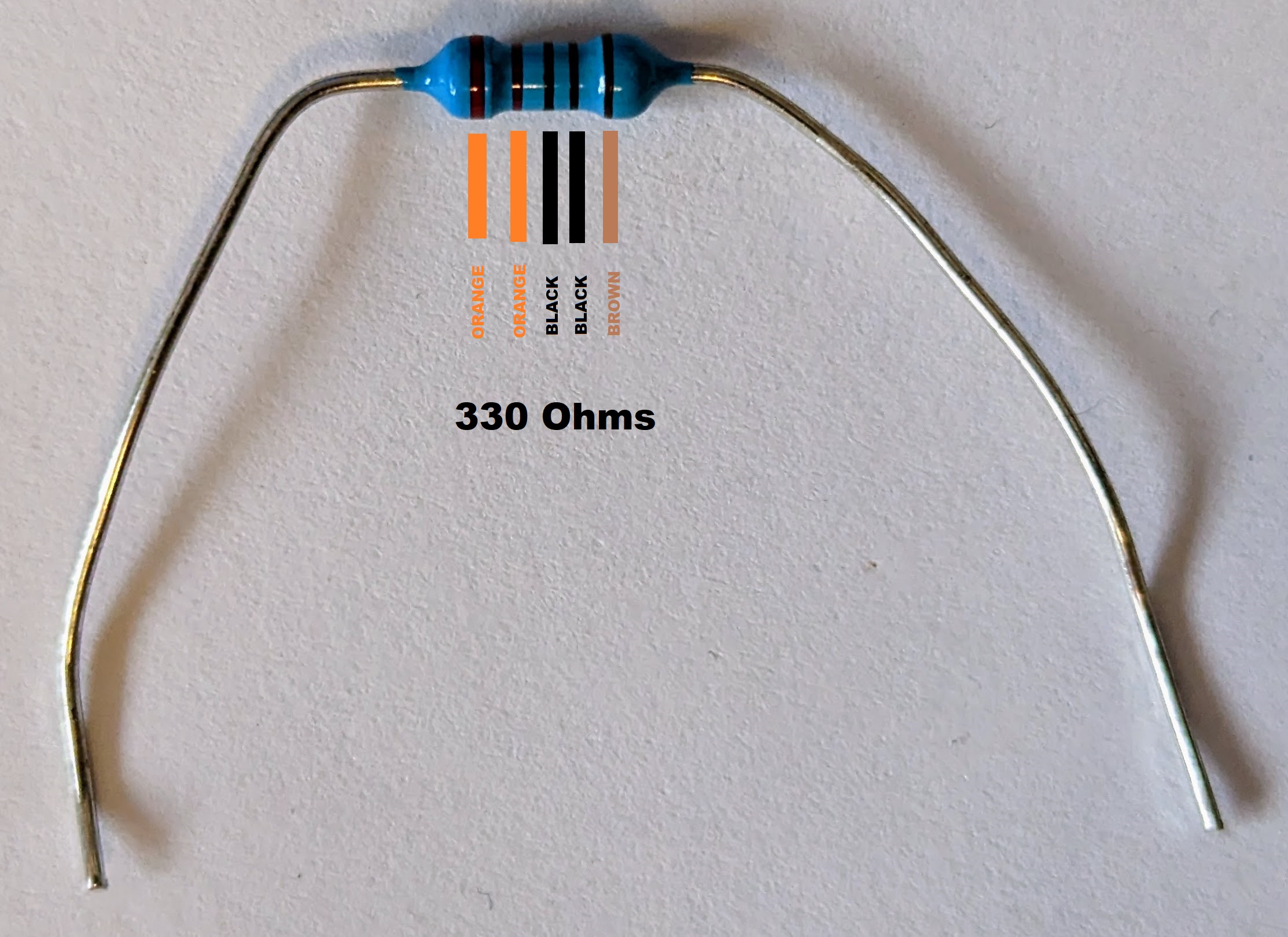
Ok, let's wire up the breadboard with the LED and resistor!
1. First, we need to connect one of the GND ("ground") pins to the negative (-) bar on the breadboard. You simply need to insert a wire into the GND pin on the board and then to any hole in the negative bar on the breadboard. In the image below, this is the black wire. This will enable us to gound our circuiut later.
2. Next, a wrie from the 13 pin of the board to a line on the bread board (A7 in this example).
3. Then connect a resistor from that line to another further down the line (E7 to E10). Don't be afraid to give yourself some room.
4. Next, connect the positive (+) side of your LED to the resistor, through the breadboard, by inserting the long leg of the LED into another hole on the same row you just connected the resistor. The negative (-) side of the LED will be put directly into the negative (-) bar on the breadboard. When you are done, your setup should look like the picture below.
<br><br>
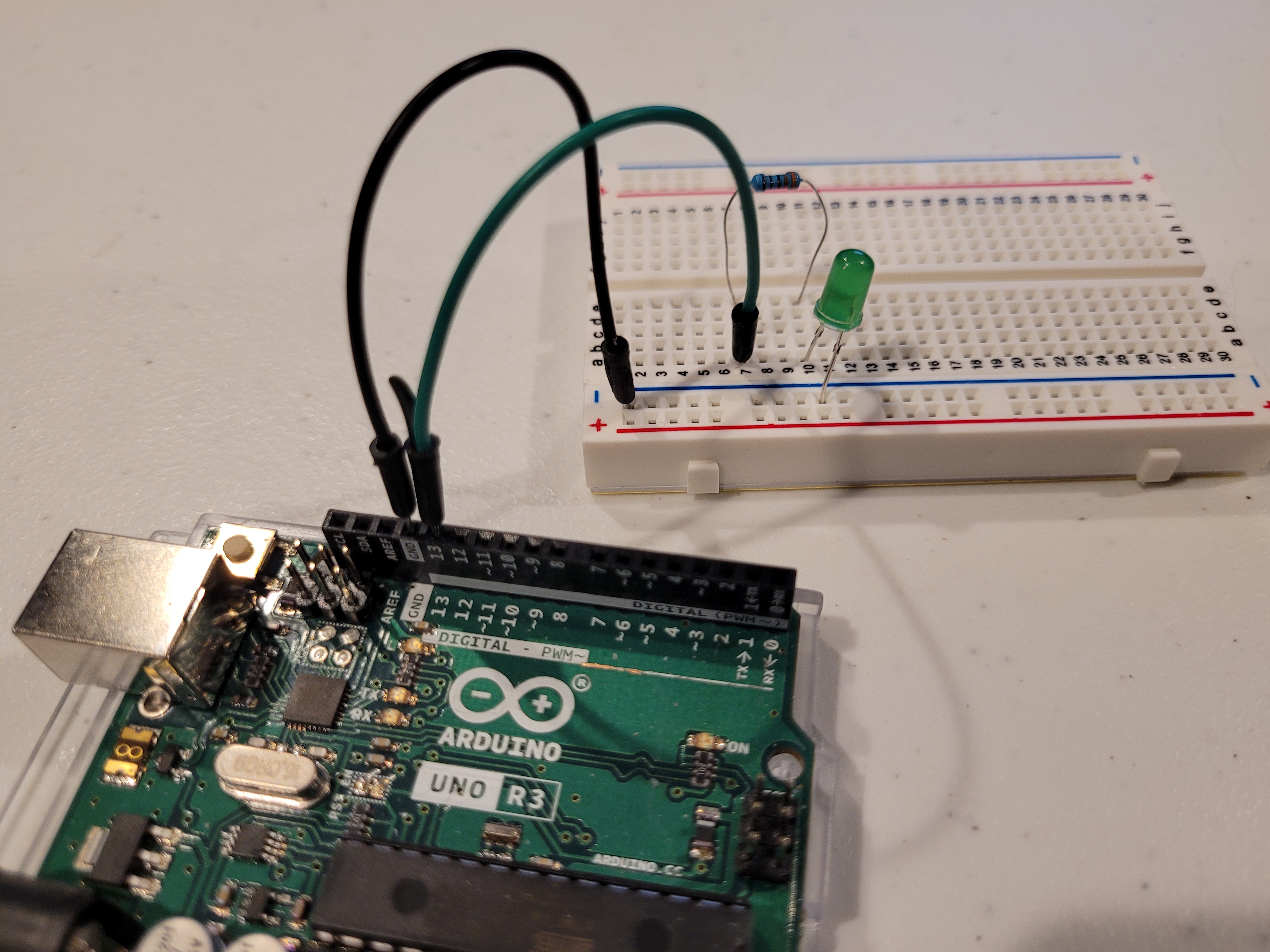
#### Working project
- Copy and paste this code into your sketch and run it
```java
void setup()
{
// Green LED
pinMode(13,OUTPUT);
}
void loop()
{
// Blink your LED
digitalWrite(13, HIGH);
delay(500);
digitalWrite(13, LOW);
delay(500);
}
Congratulations, you have created your first complete circuit!! In the next step, we’ll write some code and wire up more LEDs to blink in squence.
Using the same principles you learned above, wire two more LEDs, connecting them to PIN 12 and PIN 11. Give yourself plenty of room when placeing the new LEDs. The image below is what the final circuit should look like. Be careful that none of the resistor or LED wires are touching each other, it can get pretty crowded on the breadboard if you are not careful.
void loop()
{
// Cycle through the LEDs and blink them
digitalWrite(13, HIGH);
delay(500);
digitalWrite(13, LOW);
delay(500);
digitalWrite(12, HIGH);
delay(500);
digitalWrite(12, LOW);
delay(500);
digitalWrite(11, HIGH);
delay(500);
digitalWrite(11, LOW);
delay(500);
}
```
Congratulations! You have successfully looped a multi LED circut.
Watch the walk-through video for guidance!